How to Process Unstructured PDFs with Python in 2025
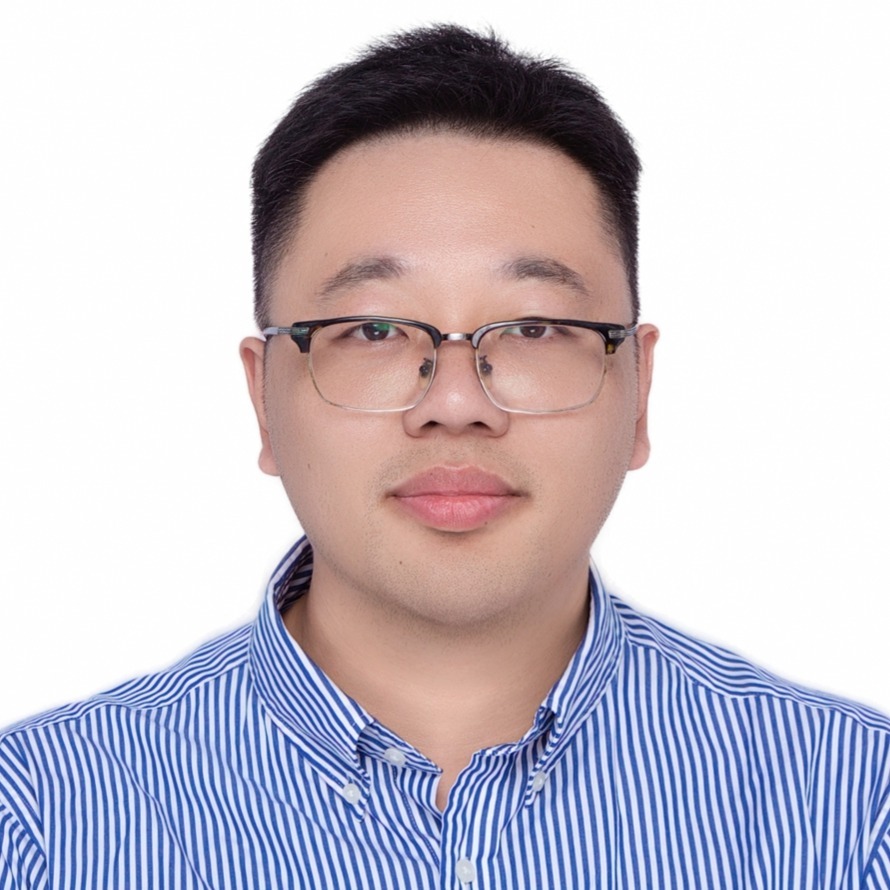
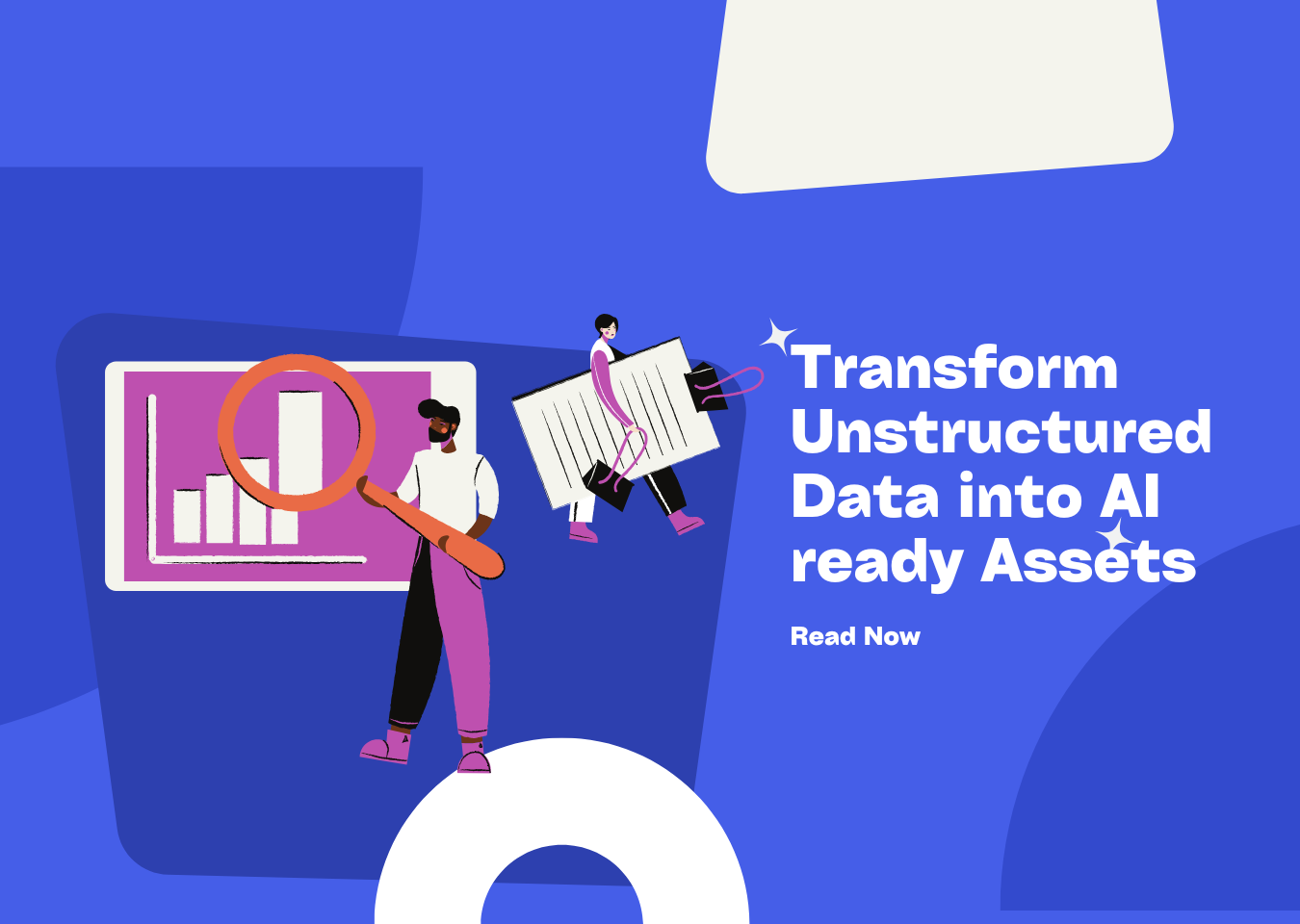
Python continues to dominate as the top choice for processing unstructured PDFs in 2025. Its versatility and robust ecosystem make it indispensable for handling complex document structures. Libraries like PyPDF2, PyMuPDF, and Tesseract simplify text extraction, while the Unstructured library excels at managing enterprise-level data. These tools reduce preprocessing time, allowing you to focus on analysis. For instance, Tesseract converts images to text, PyPDF2 extracts and manipulates PDF content, and PyMuPDF handles annotations and metadata. Whether you work with scanned documents or multi-column layouts, Python equips you with the tools to extract meaningful insights from any unstructured pdf.
Key Takeaways
-
Python is great for working with unstructured PDFs. It has strong tools like PyPDF2, PyMuPDF, and Tesseract.
-
Setting up Python is important. Install Python, pip, and needed tools to work with PDFs.
-
Use PyPDF2 to pull out simple text. Use PyMuPDF for harder tasks like notes and file details.
-
For scanned PDFs, Tesseract OCR is a must. Fix images first to make text clearer for better results.
-
Save time by writing scripts to process many PDFs at once. This makes work faster and easier.
Setting Up Your Python Environment
Before you can process unstructured PDFs, you need to set up your Python environment. This involves installing Python, libraries, and tools like Tesseract for OCR functionality. Follow these steps to get started.
Installing Python and Libraries
Installing Python and pip
First, ensure Python is installed on your system. Download the latest version from python.org. During installation, check the box to add Python to your system’s PATH. This step simplifies running Python commands from the terminal. After installation, verify it by running the command:
python --version
Next, ensure pip, Python’s package manager, is installed. Most Python installations include pip by default. Confirm its presence with:
pip --version
Installing libraries like unstructured
, PyPDF2
, and PyMuPDF
Once Python and pip are ready, install the required libraries. For example, to install the unstructured
library, use the following command:
pip install "unstructured[all-docs]"
This library is excellent for handling unstructured pdf files. Additionally, install PyPDF2
and PyMuPDF
for text extraction and metadata handling:
pip install PyPDF2 PyMuPDF
These libraries provide robust tools for parsing and processing PDFs.
Setting Up OCR with Tesseract
Installing Tesseract on your system
Scanned PDFs often require OCR to extract text. Install Tesseract OCR based on your operating system:
-
For macOS:
brew install tesseract
-
For Ubuntu:
sudo apt install tesseract-ocr
-
For Windows: Follow the official instructions from the Tesseract team.
Verify the installation by running:
tesseract -v
Integrating Tesseract with Python using pytesseract
To use Tesseract in Python, install the pytesseract
library:
pip install pytesseract
You also need the Pillow
library for image processing:
pip install Pillow
With these tools, you can extract text from images embedded in PDFs.
Verifying the Environment
Running a test script to ensure all tools and libraries are working
Before diving into PDF processing, confirm that your environment is set up correctly. Create a virtual environment to isolate dependencies:
python -m venv pdf_env
source pdf_env/bin/activate # For macOS/Linux
pdf_env\Scripts\activate # For Windows
Install all required libraries within this environment. Then, write a simple script to test the setup:
import PyPDF2
import pytesseract
from PIL import Image
print("Environment setup successful!")
Run the script. If no errors occur, your environment is ready for processing unstructured pdf files.
Tip: Use a
requirements.txt
file to manage dependencies. This ensures consistency across installations.
Extracting Text and Data from Unstructured PDFs
Using PyPDF2 for Basic Text Extraction
Reading and parsing PDF files
PyPDF2 is a reliable library for reading and parsing PDF files. Start by opening a PDF file in read-binary mode. Use PyPDF2’s PdfReader
class to load the file. This class allows you to access the document’s structure and content. For example:
from PyPDF2 import PdfReader
reader = PdfReader("example.pdf")
print(f"Number of pages: {len(reader.pages)}")
This code reads the PDF and displays the total number of pages.
Extracting text from individual pages
To extract text, loop through the pages using the pages
attribute. Use the extract_text()
method to retrieve text from each page. Here’s a sample script:
for page in reader.pages:
print(page.extract_text())
PyPDF2 works well for basic text extraction but may struggle with complex layouts in unstructured pdf files.
Using PyMuPDF for Enhanced Text Extraction
Extracting text with formatting and annotations
PyMuPDF, also known as Fitz, offers advanced features for extracting text with formatting and annotations. It processes PDFs faster and supports multiple file formats like XPS and CBZ. Use the get_text()
method to extract text while preserving formatting. For example:
import fitz # PyMuPDF
doc = fitz.open("example.pdf")
for page in doc:
print(page.get_text("text"))
This method ensures better accuracy when dealing with complex layouts.
Handling embedded images and metadata
PyMuPDF excels at handling embedded images and metadata. Use the get_images()
method to extract images and the metadata
attribute to retrieve document properties. Its speed and efficiency make it ideal for processing large unstructured pdf files.
Using Tesseract for Scanned PDFs
Converting scanned PDFs to images
Scanned PDFs require conversion to images before text extraction. Use libraries like PyMuPDF or Pillow to convert each page into an image. For example:
from PIL import Image
import fitz
doc = fitz.open("scanned.pdf")
for page_num in range(len(doc)):
pix = doc[page_num].get_pixmap()
pix.save(f"page_{page_num}.png")
Performing OCR to extract text from images
Tesseract performs OCR on images to extract text. Follow these steps for optimal results:
-
Apply binarization to remove color noise.
-
Reduce noise to eliminate small imperfections.
-
Realign tilted text to improve recognition accuracy.
Use the pytesseract.image_to_string()
method to extract text:
import pytesseract
from PIL import Image
text = pytesseract.image_to_string(Image.open("page_0.png"))
print(text)
Tesseract achieves high accuracy when these preprocessing steps are applied.
Handling Complex Document Structures in Unstructured PDFs
Image Source: unsplash
Extracting Data from Multi-Column Layouts
Identifying and isolating column structures
Multi-column layouts in PDFs can be challenging to process due to their complex structures. You may encounter issues like overlapping text or misaligned columns. To address these, use advanced PDF parsers or machine learning models that can identify and isolate column structures effectively. Pre-processing steps, such as converting the PDF to a simpler format, can also help. Here’s a quick overview of common challenges and solutions:
Challenge | Solution |
---|---|
Handling Complex PDF Structures | Use machine learning models and sophisticated PDF parsers to navigate complex layouts. |
Ensuring Data Quality | Implement pre-processing steps and post-extraction validation workflows to maintain quality. |
Software and Tool Integration | Ensure compatibility with various output formats and provide robust APIs for integration. |
Extracting text from specific columns
Once you isolate the columns, extract text from each column individually. Libraries like PyMuPDF allow you to define bounding boxes for specific areas of a page. For example:
rect = fitz.Rect(50, 50, 300, 800) # Define the column area
text = page.get_text("text", clip=rect)
print(text)
This method ensures you extract text only from the desired column, improving accuracy.
Extracting Tables from PDFs
Using libraries like camelot
or tabula
for table extraction
Tables in PDFs often contain structured data that you need to extract. Libraries like camelot
and tabula
are excellent choices for this task. Camelot works best with PDFs that have clearly defined table borders, while Tabula handles scanned PDFs effectively. Here’s a comparison of popular libraries:
Library | Ease of use | Accuracy | Output Structure | Notes |
---|---|---|---|---|
LLMWhisperer | Very high | Very high | Very high | Excellent for complex tables; Built-in support for OCR; Direct LLM input |
Pdfplumber | Medium | Very High | Very High | Excellent for complex tables; detailed control |
Pdftables | Very High | High | High | Requires API key; very user-friendly |
Exporting tables to structured formats like CSV
After extracting tables, export them to structured formats like CSV for further analysis. Camelot provides a simple method to save tables:
import camelot
tables = camelot.read_pdf("example.pdf")
tables[0].to_csv("output.csv")
This approach ensures your data is ready for use in spreadsheets or databases.
Managing Mixed Content PDFs
Separating text, images, and tables
Mixed content PDFs often combine text, images, and tables, making extraction more complex. To manage this, use tools like PyMuPDF to separate content types. For example, extract images using the get_images()
method and text using get_text()
. Simplify tables to enhance accessibility and ensure all graphics include ALT text for compliance.
Combining extracted data into a structured format
Once you extract the components, combine them into a structured format like JSON or a database. This step ensures the data is easy to analyze and integrate with other systems. Use Python libraries like Pandas to organize the extracted data efficiently.
Tip: Avoid using images of text whenever possible. Text-based content improves readability and accessibility for screen readers.
Advanced Features and Customizations
Extracting Specific Text Blocks
Using regular expressions for targeted text extraction
Regular expressions (regex) allow you to extract specific text patterns from unstructured PDFs. They are particularly effective for validating and isolating data formats. For example, you can use regex to ensure email addresses, phone numbers, or postal codes match expected patterns. Regex also helps you extract relevant content from larger text blocks. For instance, you can isolate customer feedback while removing unwanted text or HTML tags. Here’s a simple example of extracting email addresses:
import re
text = "Contact us at support@example.com or sales@example.org."
emails = re.findall(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b', text)
print(emails)
This script identifies and extracts all email addresses from the given text.
Filtering text based on keywords or patterns
You can filter text by searching for specific keywords or patterns. This approach is useful when you need to extract only relevant sections of a document. For example, you might search for terms like “invoice” or “total” in financial documents. Use Python’s re
module to implement keyword-based filtering efficiently.
Automating PDF Processing
Writing scripts to process multiple PDFs in bulk
Automating PDF processing saves time when working with large datasets. Write scripts to process multiple PDFs in a single run. Use libraries like os
to iterate through files in a directory and apply extraction functions to each file. Here’s an example:
import os
from PyPDF2 import PdfReader
directory = "pdf_folder"
for filename in os.listdir(directory):
if filename.endswith(".pdf"):
reader = PdfReader(os.path.join(directory, filename))
for page in reader.pages:
print(page.extract_text())
This script processes all PDFs in a folder and extracts their text.
Scheduling tasks with Python automation tools
Schedule your PDF processing tasks using tools like schedule
or APScheduler
. Key considerations include data extraction accuracy, handling complex layouts, and ensuring scalability. Choose libraries that suit your needs to improve efficiency and reliability.
Exporting and Visualizing Extracted Data
Saving data to formats like CSV, JSON, or databases
After extracting data, save it in structured formats like CSV, JSON, or databases for further analysis. Use Python’s csv
or json
modules to export data. For example, save extracted text to a CSV file:
import csv
data = [["Page", "Content"], [1, "Sample text"]]
with open("output.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerows(data)
This ensures your data is ready for integration with other tools.
Visualizing data using Python libraries like Matplotlib or Seaborn
Visualize extracted data to uncover trends and insights. Use libraries like Matplotlib or Seaborn to create charts and graphs. For instance, plot the frequency of keywords in a document:
import matplotlib.pyplot as plt
keywords = ["invoice", "total", "amount"]
counts = [10, 5, 8]
plt.bar(keywords, counts)
plt.xlabel("Keywords")
plt.ylabel("Frequency")
plt.title("Keyword Frequency in Document")
plt.show()
Visualizations make your data more accessible and actionable.
Applying Extracted Data Effectively
Real-World Use Cases
Analyzing business reports and financial documents
Extracted data from unstructured pdf files can transform how you handle business reports and financial documents. By automating data extraction, you can quickly analyze key metrics like revenue, expenses, and profit margins. This approach saves time and reduces errors compared to manual methods. For example, businesses often process financial reports, legal documents, and healthcare records to gain insights and make informed decisions.
Legal assessments firms have also benefited from automated data extraction. One firm improved its process for analyzing mental health questionnaires. This allowed them to evaluate crime probabilities faster and with greater accuracy. These examples highlight how extracted data can streamline operations and enhance decision-making in various industries.
Extracting insights from academic research papers
Academic research papers often contain valuable information buried in complex layouts. Extracting data from these documents enables you to analyze trends, compare findings, and identify gaps in research. For instance, scientific papers often include tables, graphs, and multi-column text. By using Python tools, you can extract and organize this data for further study. This capability is especially useful for researchers who need to review large volumes of literature efficiently.
Integrating with Other Tools and Systems
Using APIs to send data to external platforms
Once you extract data, you can integrate it with other tools and systems using APIs. APIs allow you to send extracted data to platforms like CRMs, data visualization tools, or cloud storage. For example, you can automate the transfer of financial data to accounting software or upload extracted tables to a database for analysis. This integration ensures seamless workflows and reduces manual intervention.
Combining extracted data with machine learning models for analysis
Integrating extracted data with machine learning models unlocks advanced analytical capabilities. Machine learning optimizes document processing by speeding up data handling and reducing costs by up to 60%. It eliminates the need for manual data extraction, saving time and effort. Machine learning also improves accuracy by identifying synonyms and related terms, ensuring more reliable results. Businesses benefit from quicker access to critical data, enabling better decision-making and improved customer satisfaction. For example, you can use extracted data to train models that predict trends or classify documents based on content.
Tip: Always validate your extracted data before integrating it with other systems to ensure accuracy and consistency.
📖See Also
Subscribe to Our Newsletter
Get the latest updates and exclusive content delivered straight to your inbox