Mastering RAG Optimization: The Ultimate Guide to Unstructured Document Parsing
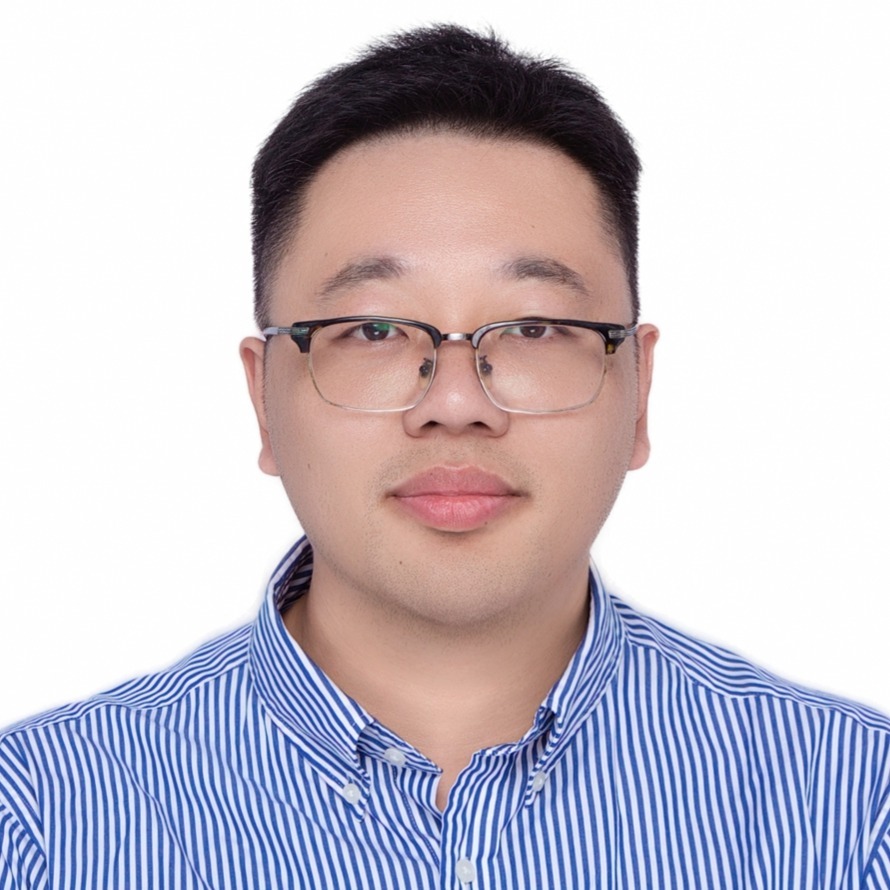
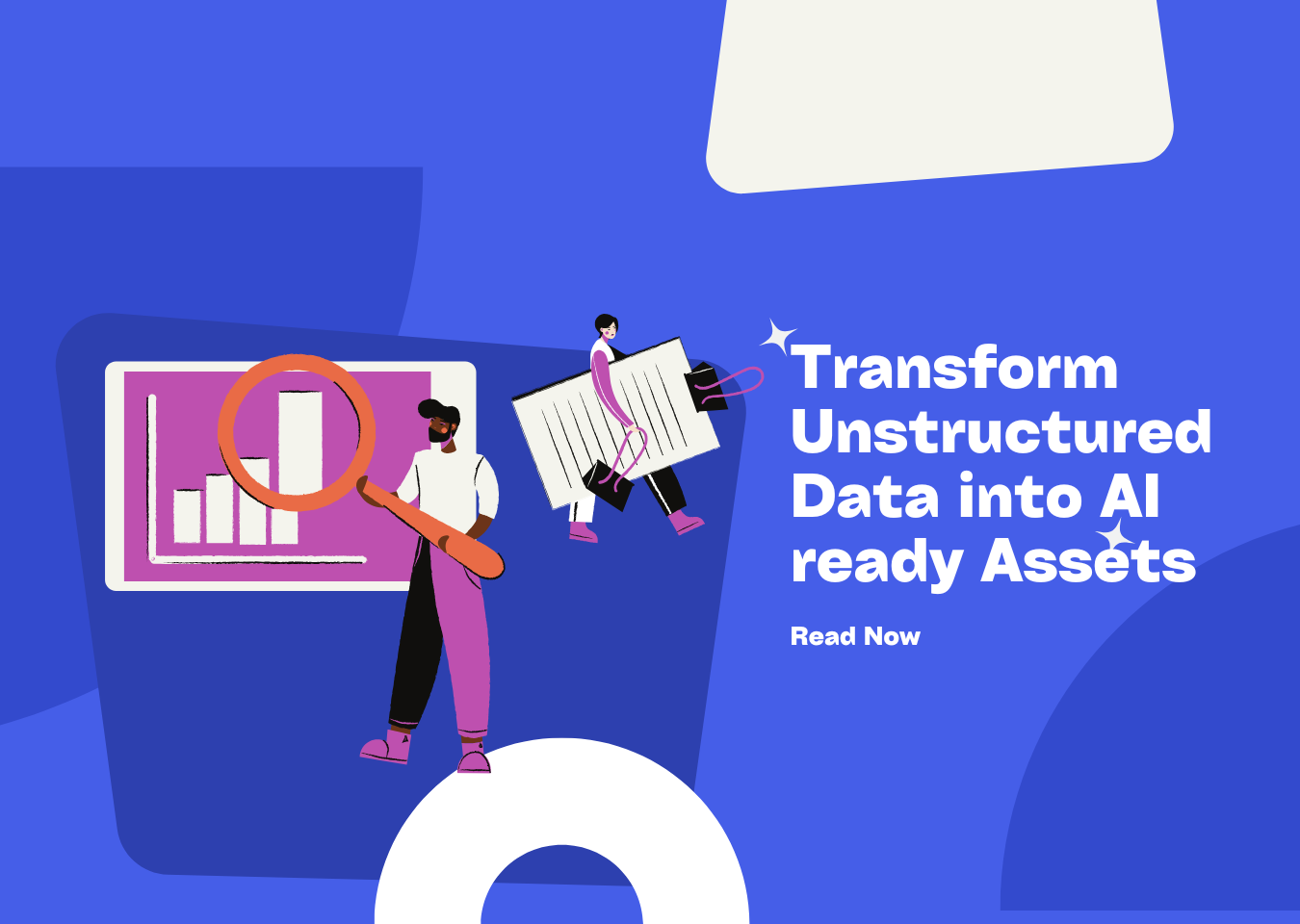
I. Introduction
RAG (Retrieval-Augmented Generation), proposed by the Facebook AI Research (FAIR) team in 2020, is a cutting-edge artificial intelligence technology. It ingeniously combines the retrieval and generation processes. By retrieving relevant information from vast amounts of data, it assists language models in producing more accurate and detailed text content. RAG technology is highly regarded mainly due to the following advantages:
- Utilization of external knowledge bases: It can introduce a wider range of knowledge sources and provide in-depth and accurate answers.
- Timeliness of knowledge update: It enables dynamic knowledge updates without the need to retrain the model.
- Explainability of generated answers: The answers directly reference the retrieved materials, enhancing the transparency and credibility of the responses.
RAG technology has a wide range of applications, including natural language processing tasks such as question-answering systems, document generation, intelligent assistants, information retrieval, and knowledge graph filling. It significantly improves the performance of large language models in handling knowledge-intensive tasks.
There are various ways to optimize RAG technology, including knowledge base processing, word vector models, retrieval algorithms, reranking algorithms, and inference generation. This article will focus on the detailed optimization work based on knowledge base parsing.
II. Parsing Methods
2.1 TXT Document Parsing
Use the UnstructuredFileLoader class to load TXT files and extract their contents.
from langchain.document_loaders import UnstructuredFileLoader
loader = UnstructuredFileLoader("./test/test_file1.txt")
docs = loader.load()
print(docs[0].page_content[:400])
2.2 Word Document Parsing
Load and parse Word documents through the UnstructuredWordDocumentLoader class.
from langchain.document_loaders import UnstructuredWordDocumentLoader
loader = UnstructuredWordDocumentLoader("example_data/fake.docx")
data = loader.load()
print(data)
2.3 PDF Document Parsing
There are multiple ways to parse PDF documents:
2.3.1 Based on the unstructured library
First, you need to install OCR-related function libraries to parse PDF documents.
from langchain.document_loaders import UnstructuredFileLoader
loader = UnstructuredFileLoader("./example_data/layout-parser-paper.pdf", mode="elements")
docs = loader.load()
print(docs[:5])
2.3.2 PyPDF Tool
Use the PyPDF library to install and retrieve PDF documents by page number.
from langchain.document_loaders import PyPDFLoader
loader = PyPDFLoader("example_data/layout-parser-paper.pdf")
pages = loader.load_and_split()
2.3.3 Online Reading Tools
The method of loading PDF documents online.
from langchain.document_loaders import OnlinePDFLoader
loader = OnlinePDFLoader("https://arxiv.org/pdf/2302.03803.pdf")
data = loader.load()
print(data)
2.3.4 PDFMiner
Use the PDFMiner library to load PDF documents.
from langchain.document_loaders import PDFMinerLoader
loader = PDFMinerLoader("example_data/layout-parser-paper.pdf")
data = loader.load()
2.4 Email Parsing
Use the UnstructuredEmailLoader class to load and parse email data.
from langchain.document_loaders import UnstructuredEmailLoader
loader = UnstructuredEmailLoader('example_data/fake-email.eml')
data = loader.load()
2.5 Image Content Parsing
Process image formats such as JPG and PNG and convert them into the document data format required for downstream RAG tasks.
from langchain.document_loaders.image import UnstructuredImageLoader
loader = UnstructuredImageLoader("layout-parser-paper-fast.jpg")
data = loader.load()
2.6 Markdown Content Parsing
When parsing Markdown files, special attention should be paid to setting the mode and autodetect_encoding parameters.
loader = document_loaders.UnstructuredFileLoader(filepath, mode="elements", autodetect_encoding=True)
docs = loader.load()
2.7 PPT Content Parsing
Load and parse PPT documents.
from langchain.document_loaders import UnstructuredPowerPointLoader
loader = UnstructuredPowerPointLoader("example_data/fake-power-point.pptx")
data = loader.load()
2.8 DeepDoc Parsing
DeepDoc is a component in the RAGFlow framework and supports multiple text slicing templates to adapt to different business scenarios.
Link to the RAGFlow framework: RAGFlow on GitHub
Through these methods, document contents in different formats can be efficiently parsed into structured data, which can then play its role in RAG technology and improve the accuracy and efficiency of information retrieval and text generation.
PDF Parsing Optimization Methods
-
Use Efficient Libraries:
Choose high-performance libraries, such as PyMuPDF (also known as fitz) or PDFMiner, which can accelerate the parsing speed and improve the parsing quality.
-
Parallel Processing:
Utilize multi-threading or multi-processing to parse different parts of PDFs in parallel, especially when dealing with large or multiple PDF files.
-
Optimize OCR:
If OCR technology is required to parse image or scanned PDF documents, choose an efficient OCR engine, such as Tesseract, and adjust its parameters.
-
Choose the Appropriate Parsing Mode:
Select the parsing mode according to requirements, such as text extraction, layout analysis, or element-level parsing.
-
Cache Mechanism:
Implement a caching strategy for frequently accessed PDF file contents to avoid repeated parsing.
-
Resource Limitation:
In a resource-constrained environment, optimize the use of memory and CPU, for example, by adjusting the configuration of the parsing library.
-
Error Handling:
Enhance error handling capabilities to ensure that when parsing damaged PDF files or encountering errors, the entire processing flow will not be affected.
Chunk Processing Strategies
-
Reasonable Chunk Division:
Divide chunks reasonably according to the logical structure of the content, such as by paragraph, page, or chapter.
-
Noise Removal:
Clean up the noise data that may exist in chunks, such as irrelevant headers, footers, and page numbers.
-
Content Rearrangement:
Rearrange or format the content of chunks as necessary to meet the requirements of downstream tasks.
-
Feature Extraction:
Extract useful features from chunks, such as keywords, entities, and abstracts, for further analysis.
-
Context Preservation:
When processing chunks, maintain the context information of the text to facilitate better semantic understanding.
-
Data Enhancement:
Improve the generalization ability of the model by performing data enhancement on chunks, such as synonym replacement and sentence reorganization.
-
Index Construction:
Build indexes for chunks to facilitate quick retrieval and similarity search.
-
Multimodal Fusion:
If PDFs contain images or tables, fuse these multimodal data with text data to provide richer information.
-
Quality Assessment:
Evaluate the quality of processed chunks to ensure that they meet the requirements of subsequent applications.
-
Security Considerations:
Pay attention to data security and privacy during the processing to avoid the leakage of sensitive information.
Through the above methods and strategies, the PDF parsing process can be effectively optimized, and the parsed data can be efficiently processed to support various application scenarios.
📖See Also
- Leveraging-UnDatasio-and-deepseek-to-Analyze-Tesla-Gen-Report-A-Step-by-Step-Guide
- Leveraging-UnDatasio-and-DeepSeek-to-Analyze-Tesla-Gen-Report-2-Intelligent-Question-Answering-Unveiled
- In-Depth-Analysis-of-API-Services-Graphlit-LlamaParse-UndatasIO-etc-for-Extracting-Complex-PDF-Tables-to-Markdown
- Improving-the-Response-Quality-of-RAG-Systems-High-Quality-Enterprise-Document-Parsing
- Improving-the-Response-Quality-of-RAG-Systems-Excel-and-TXT-Document-Parsing
- How-UnDatasIO-Transforms-Unstructured-Data-for-AI-and-LLM-Success
- How-to-Use-undatasio-to-Parse-Complex-Table-Take-IRS-1040-Table-as-an-example
Subscribe to Our Newsletter
Get the latest updates and exclusive content delivered straight to your inbox