Top Tips for Extracting Table Data from HTML Efficiently
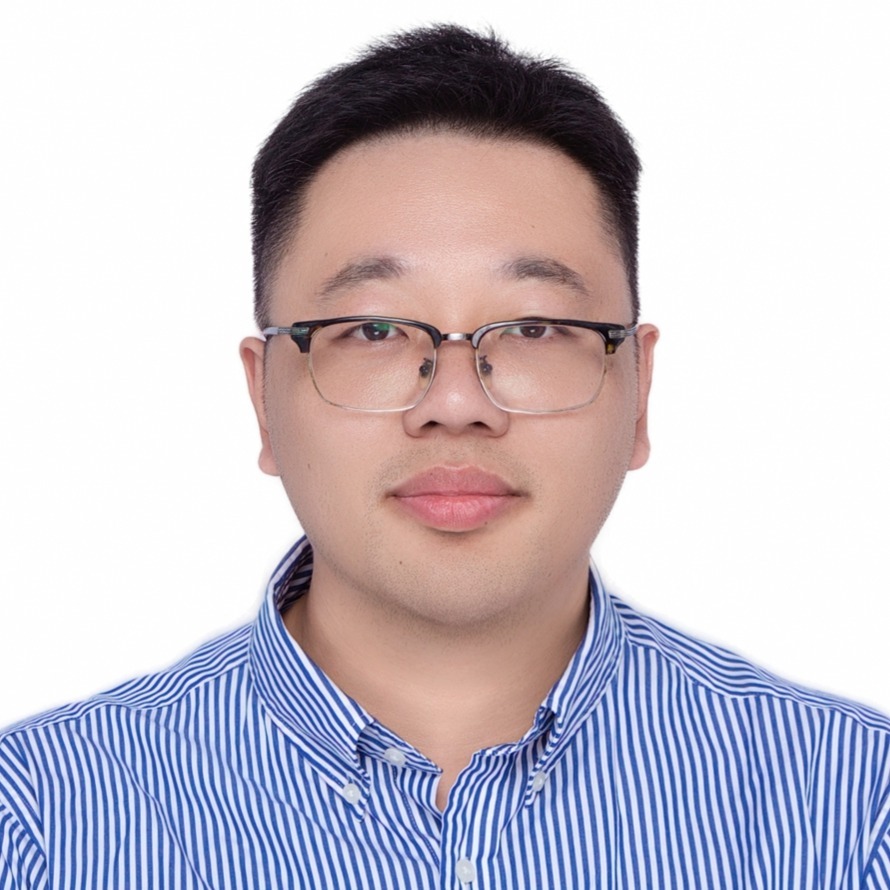
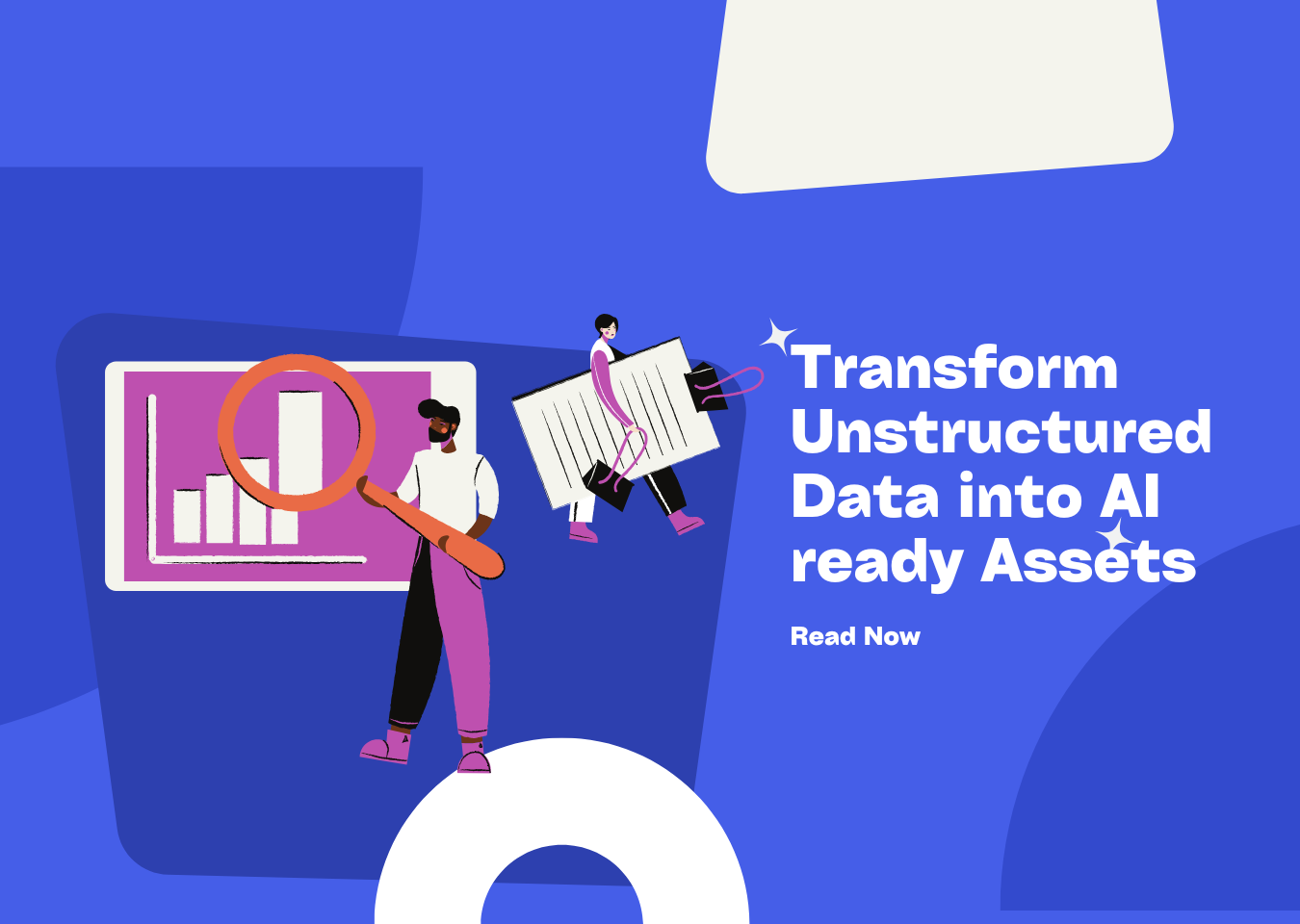
Extracting table data from HTML can feel like a daunting task, but it doesn’t have to be. Whether you’re analyzing data, creating reports, or automating workflows, knowing how to extract table data from HTML efficiently saves you time and headaches. The right tools make all the difference. From coding libraries to no-code platforms, there’s something for everyone. By streamlining the process to extract table data from HTML, you reduce errors and focus on what matters most—getting accurate results. Ready to dive in and make your workflow smoother? Let’s get started!
Key Takeaways
-
Pick the best tool for your task. Tools like BeautifulSoup, Selenium, and no-code tools like Octoparse work for different skills and needs.
-
Use automation to save time and stay consistent. Try APIs, OCR tools, and schedules to make data collection easier.
-
Fix and organize your data for better results. Remove repeats, fill missing spots, and make formats the same for accuracy.
-
Use XPath and regular expressions to get exact data. These tools help you find patterns and work with tricky HTML.
-
Try different scraping methods to see what fits you. Each tool has special features to improve your data collection.
Overview of Popular Tools
When it comes to extracting table data from HTML, choosing the right tools can make your life much easier. Let’s explore some popular options that cater to different needs and skill levels.
BeautifulSoup
BeautifulSoup is a fantastic library for parsing HTML and XML documents. It simplifies the process of navigating and extracting data from HTML tables. You can use methods like find
and find_all
to locate specific elements. Once you extract the data, you can integrate it with pandas to store and analyze it in a structured format.
Here’s a quick example to show how BeautifulSoup works:
from bs4 import BeautifulSoup
html = """<table><tr><th>Name</th><th>Age</th></tr><tr><td>John</td><td>30</td></tr></table>"""
soup = BeautifulSoup(html, 'html.parser')
# Find the table element
table = soup.find('table')
# Extract the data from the cells
data = []
for row in table.find_all('tr'):
cols = row.find_all(['td', 'th'])
cols = [ele.text.strip() for ele in cols]
data.append(cols)
print(data)
This library is perfect if you’re comfortable with Python and want precise control over your data extraction process.
Selenium
Selenium is a powerful tool for web scraping, especially when dealing with dynamic content. Many websites use JavaScript to load table data, which can be tricky to handle. Selenium solves this by simulating a browser and rendering the page fully before you extract the data.
Here’s how Selenium handles dynamic content:
-
Load the webpage using Selenium to ensure all JavaScript-rendered content is visible.
-
Retrieve the page source after the content has loaded.
-
Use a parsing library like BeautifulSoup to extract data from HTML tables.
Selenium is ideal if you’re working with websites that rely heavily on JavaScript. It gives you the flexibility to interact with the page, click buttons, or even scroll to load more data.
No-Code Tools (e.g., Octoparse)
Not a fan of coding? No problem! No-code tools like Octoparse are here to save the day. These tools are designed for users without technical expertise, making them accessible to everyone.
Here’s why you might love them:
-
They’re easy to use, thanks to their intuitive interfaces.
-
They handle complex tasks, including AJAX and JavaScript-based content.
-
You don’t need to write a single line of code to extract data from HTML tables.
Octoparse, for example, lets you visually select the table data you want to extract. Once you’ve set up your workflow, you can export the data in formats like CSV or Excel. It’s a great option if you’re looking for a quick and hassle-free solution.
XPath and Regular Expressions
When it comes to precision in extracting data, XPath and regular expressions are your go-to tools. They might sound intimidating at first, but once you get the hang of them, they’re incredibly powerful.
XPath: A Path to Precision
XPath (XML Path Language) helps you navigate through the structure of an HTML document. It’s like a GPS for locating specific elements on a webpage. If you’re dealing with complex HTML tables, XPath can pinpoint the exact rows, columns, or cells you need.
Here’s a quick example:
from lxml import html
html_content = """<table><tr><td>John</td><td>30</td></tr></table>"""
tree = html.fromstring(html_content)
# Use XPath to extract the first cell
data = tree.xpath('//table/tr/td[1]/text()')
print(data) # Output: ['John']
You can use XPath with libraries like lxml or Selenium. It’s perfect for situations where you need to extract data from HTML tables with precision.
Regular Expressions: The Pattern Matcher
Regular expressions (regex) are all about finding patterns in text. They’re great for extracting specific pieces of information, like dates, numbers, or email addresses, from messy HTML.
For example, let’s say you want to extract numbers from a table:
import re
html = "<td>Age: 30</td>"
pattern = r'\d+' # Matches one or more digits
# Find all matches
numbers = re.findall(pattern, html)
print(numbers) # Output: ['30']
Regex works well when the data you need follows a consistent pattern. However, it’s not ideal for parsing entire tables.
When to Use These Tools
Use XPath when you need to navigate structured HTML. Turn to regex for extracting specific patterns. Combining these tools with web scraping libraries can make your workflow more efficient. Whether you’re trying to extract table data from HTML or handle dynamic content, these methods give you the control you need.
Step-by-Step Guide to Extract Table Data from HTML
Image Source: pexels
Extracting Data with BeautifulSoup
Setting up BeautifulSoup
Before you can start extracting an HTML table, you need to set up BeautifulSoup. Here’s how you can do it:
-
Install BeautifulSoup and pandas using pip:
$ pip install beautifulsoup4 pandas
-
Install the requests library to fetch webpage content:
$ pip install requests
-
Use requests to retrieve the HTML content of the webpage:
import requests url = 'https://www.w3schools.com/html/html_tables.asp' html = requests.get(url).text
-
Parse the HTML content with BeautifulSoup:
from bs4 import BeautifulSoup soup = BeautifulSoup(html, 'html.parser')
Once you’ve completed these steps, you’re ready to parse HTML tables and extract data.
Parsing HTML Tables
BeautifulSoup makes it easy to extract data from HTML tables. Use the find
or find_all
methods to locate table elements. Then, loop through rows and cells to collect the data. Here’s an example:
table = soup.find('table')
data = []
for row in table.find_all('tr'):
cols = row.find_all(['td', 'th'])
data.append([col.text.strip() for col in cols])
print(data)
This approach gives you full control over how you extract table data from HTML.
Using pandas for HTML Table Extraction
Reading HTML Tables with pandas
If you’re looking for a simpler way to extract data from HTML tables, pandas is your friend. It’s perfect for structured tables. Use the read_html
function to directly load tables into a DataFrame:
import pandas as pd
url = 'https://www.w3schools.com/html/html_tables.asp'
tables = pd.read_html(url)
print(tables[0]) # Access the first table
Pandas automatically detects and structures the table, saving you time.
Exporting Data to CSV or Excel
Once you’ve loaded the table, exporting it is a breeze. Use pandas’ built-in methods to save the data:
tables[0].to_csv('output.csv', index=False) # Save as CSV
tables[0].to_excel('output.xlsx', index=False) # Save as Excel
This makes pandas a great choice for tasks like reporting or data analysis. However, it’s less flexible than BeautifulSoup for custom scraping needs.
Automating Extraction with Selenium
Setting up Selenium for Web Scraping
Selenium is a lifesaver when dealing with dynamic content. Start by installing Selenium and a browser driver like ChromeDriver:
$ pip install selenium
Then, set up your script to load the webpage:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
html = driver.page_source
This ensures all JavaScript-rendered content is fully loaded before you extract data.
Handling Dynamic Content
Dynamic tables often require interaction, like clicking buttons or scrolling. Selenium lets you automate these actions. For example, you can click a button to load more rows:
button = driver.find_element_by_id('load-more')
button.click()
Once the content is loaded, use BeautifulSoup or pandas to parse the HTML tables. Selenium is ideal for complex web scraping projects, especially when tables have inconsistent formats or large data volumes.
Leveraging No-Code Tools
Overview of Octoparse and Similar Tools
No-code tools are a game-changer if you want to extract data from HTML tables without writing a single line of code. These tools are designed to make web scraping accessible to everyone, even if you’re not a programmer. Octoparse is one of the most popular options, but there are many others out there.
Here’s what makes no-code tools so useful:
-
They let you visually select the data you want to extract, like clicking on table rows or columns.
-
Many of them come with built-in connectors to pull data from websites, APIs, or even PDFs.
-
They simplify complex tasks, such as handling JavaScript-rendered content or integrating data into spreadsheets.
Some common use cases for no-code tools include:
-
Web scraping tools to extract table data from HTML.
-
Email scrapers for pulling addresses or message content.
-
API extractors to fetch data directly into databases.
-
PDF extractors that use OCR to grab text from scanned documents.
If you’re looking for a quick and easy way to extract table data from HTML, no-code tools like Octoparse are worth exploring.
Exporting Data from No-Code Tools
Once you’ve set up your workflow in a no-code tool, exporting the data is straightforward. Most tools allow you to save the extracted data in formats like CSV, Excel, or JSON. For example, in Octoparse, you can:
-
Run your extraction task to collect the data.
-
Choose your preferred export format.
-
Download the file to your computer or send it directly to a database.
This process makes no-code tools perfect for tasks like reporting or data analysis. You get clean, structured data without the hassle of coding.
Using XPath and Regular Expressions
Writing XPath Queries for Precise Extraction
XPath is like a treasure map for navigating HTML documents. It helps you pinpoint specific elements, making it ideal for precise data extraction. You can use it to extract data from HTML tables with incredible accuracy.
Here are some tips to optimize your XPath queries:
-
Use
starts-with()
to select elements where the text begins with a specific value. -
Try
ends-with()
for elements where the text ends with a certain value. -
Use
substring()
to grab a specific portion of text within an element. -
Apply
normalize-space()
to remove extra spaces from text nodes. -
Use
count()
to find out how many elements match your query.
For example, if you want to extract the first column of a table:
from lxml import html
html_content = """<table><tr><td>John</td><td>30</td></tr></table>"""
tree = html.fromstring(html_content)
# Extract the first column using XPath
data = tree.xpath('//table/tr/td[1]/text()')
print(data) # Output: ['John']
XPath gives you the precision you need for complex web scraping tasks.
Extracting Patterns with Regular Expressions
Regular expressions (regex) are your go-to tool for finding patterns in text. They’re especially handy when you need to extract specific data, like dates or numbers, from messy HTML.
For instance, if you want to extract ages from a table:
import re
html = "<td>Age: 30</td>"
pattern = r'\d+' # Matches one or more digits
# Find all matches
numbers = re.findall(pattern, html)
print(numbers) # Output: ['30']
Regex works best when the data you need follows a consistent pattern. Combine it with XPath for even more powerful data extraction.
Best Practices for Efficiency
Image Source: pexels
Automating Repetitive Tasks
Repetitive tasks can drain your time and energy. Automating them not only saves effort but also ensures consistency. Here are some effective methods you can use:
-
APIs: Use APIs to fetch data in real-time. This eliminates the need for manual updates.
-
OCR Technology: Extract text from images or scanned documents. It’s perfect for automating data entry.
-
Scheduling Automated Tasks: Set up schedules to run your data extraction workflows automatically. This keeps your process on track without manual intervention.
-
Robotic Process Automation (RPA): RPA tools handle repetitive tasks, freeing you up for more strategic work.
-
Data Validation Techniques: Automate checks to ensure the extracted data is accurate and formatted correctly.
If you’re into coding, tools like BeautifulSoup, Scrapy, and Puppeteer can help you automate web scraping workflows. For non-coders, Octoparse and Tabula are excellent options.
Handling Large Datasets
Working with large datasets can feel overwhelming. They demand more storage, time, and resources. Here’s how you can tackle these challenges:
-
Use cloud storage solutions to manage large files. They’re scalable and cost-effective.
-
Break the dataset into smaller chunks. This makes processing and analysis easier.
-
Choose tools designed for big data, like Apache Spark or Dask, to handle large volumes efficiently.
The larger the dataset, the higher the risk of errors. Complex formats, unstructured data, and maintaining accuracy can make the process tricky. Always double-check your results to avoid incomplete or inaccurate extractions.
Visualizing large datasets can also be tough. Specialized tools like Tableau or Power BI can help you make sense of the data.
Cleaning and Formatting Extracted Data
Raw data is rarely perfect. Cleaning and formatting it ensures you get accurate and usable results. Start by removing unnecessary characters, fixing encoding issues, and standardizing formats.
Here’s a quick checklist to clean your data:
-
Remove duplicates to avoid skewed results.
-
Handle missing values by filling them in or removing incomplete rows.
-
Convert data types (e.g., strings to numbers) for easier analysis.
-
Standardize date formats to maintain consistency.
Python libraries like pandas are great for cleaning data. For example, you can use drop_duplicates()
to remove duplicate rows or fillna()
to handle missing values. Clean data not only improves accuracy but also makes your analysis more reliable.
Optimizing Code for Speed and Accuracy
When you’re extracting table data from HTML, speed and accuracy are everything. Nobody wants to wait forever for a script to run or deal with messy, unreliable data. The good news? You can optimize your code with a few smart techniques that make your workflow faster and more precise.
Here are some tips to get you started:
-
Automate validation checks: Set up automated processes to verify data accuracy as it’s being loaded. This catches errors early and saves you from headaches later.
-
Use bulk inserts: Instead of processing one record at a time, group multiple records into a single operation. This reduces overhead and speeds up data handling.
-
Apply indexing: If you’re working with SQL databases, indexing frequently queried columns can drastically improve retrieval times.
-
Leverage parallel processing: Break large datasets into smaller chunks and process them simultaneously. This approach cuts down on processing time and keeps things running smoothly.
-
Partition your data: Divide massive datasets into manageable sections. This not only speeds up retrieval but also reduces the load on your database.
Accuracy matters just as much as speed. To ensure your data is reliable:
-
Automate extraction tasks: This minimizes human error and ensures you get consistent results every time.
-
Validate at the source: Check data for completeness and accuracy as soon as it’s extracted. It’s easier to fix issues upfront than to clean up later.
-
Audit regularly: Schedule audits to spot and fix problems in your extracted data. Use cleansing techniques to maintain high-quality results.
By combining these strategies, you’ll create a workflow that’s both fast and reliable. Whether you’re handling small tables or massive datasets, these optimizations will save you time and effort. Plus, you’ll feel confident knowing your data is accurate and ready to use. 🚀
Choosing the right tool to extract web data from HTML can make your workflow smoother and more efficient. Think about factors like ease of use, scalability, and output formats. Tools that support delays between requests or offer responsive support can also save you headaches. Don’t forget to follow best practices, like cleaning your data and automating repetitive tasks. Experiment with different scrapers to find what works best for your needs. With the right approach, you’ll import web data accurately and save valuable time.
📖See Also
- Undatas-io-2025-New-Upgrades-and-Features
- UndatasIO-Feature-Upgrade-Series1-Layout-Recognition-Enhancements
- UndatasIO-Feature-Upgrade-Series2-OCR-Multilingual-Expansion
- Undatas-io-Feature-Upgrade-Series3-Advanced-Table-Processing-Capabilities
- Undatas-io-2025-New-Upgrades-and-Features-French
- Undatas-io-2025-New-Upgrades-and-Features-Korean
Subscribe to Our Newsletter
Get the latest updates and exclusive content delivered straight to your inbox