Mastering Redis Py: A Practical Guide to Commercial Applications
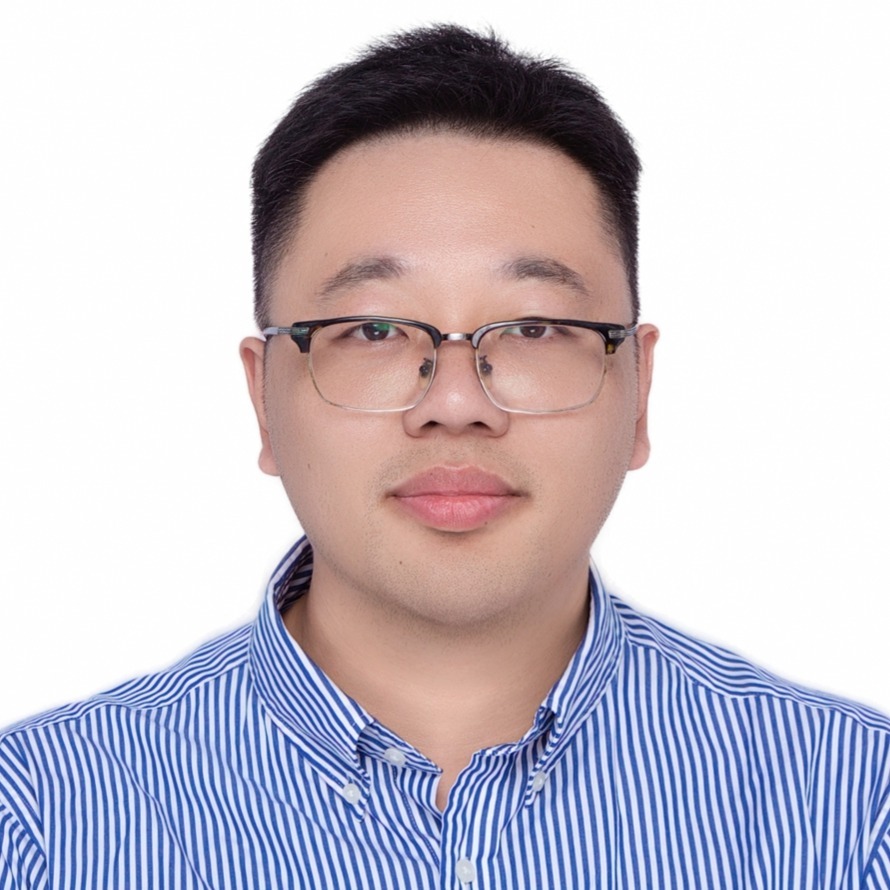
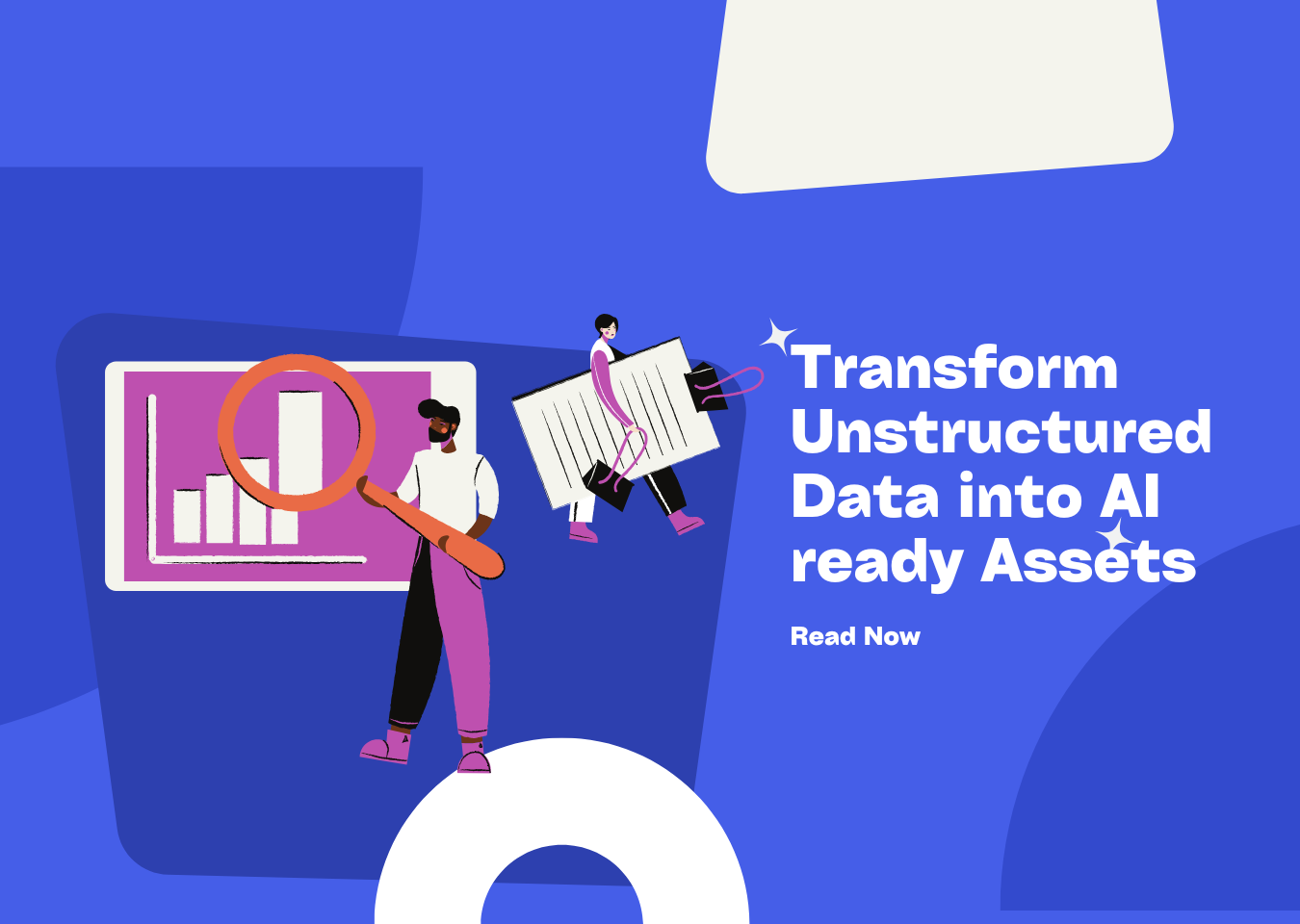
Boost Performance and Scalability in Your Python Applications with Redis Py
I. Introduction
Picture this: it’s Black Friday. Your e-commerce site, usually a well-oiled machine, is creaking, groaning, and generally having a meltdown under the stampede of bargain-hunters. Transactions are timing out, your hair is falling out, and revenue? Oh, that’s taking a nosedive. Let’s be honest, slow application performance isn’t just a minor hiccup; it’s the kind of oopsie that makes your accountant weep. In a world where ‘instant gratification’ is practically a human right, businesses need something seriously zippy to keep up.
Cue the dramatic entrance of Redis! Think of it as the superhero of in-memory data stores – a ridiculously fast cache, a real-time analytics whiz, and a whole lot more. Team it up with redis-py
, its trusty Python sidekick, and you’ve got a dynamic duo ready to turbocharge your application’s performance. Redis isn’t just another database your tech team mumbles about; it’s a secret weapon, dishing out speed and flexibility like a five-star chef in the commercial kitchen. It’s all about serving your customers at lightning speed and scaling up when the party gets wild.
Now, a little wrinkle in the red carpet: the world of tech licensing recently threw a bit of a curveball with Redis, leading to new kids on the block like Valkey popping up. So, picking the right tool for your treasure chest is more important than ever. Don’t you worry, though! This guide is your treasure map to navigating these choices and unlocking the awesome power of redis-py
.
II. What is Redis and Why Use It With Python?
So, what’s the big deal with Redis? Well, imagine a database that lives on a caffeine drip – that’s Redis for you. It’s an in-memory data structure store, which is a fancy way of saying it keeps data in your computer’s super-fast RAM instead of slogging through traditional disk-based databases. The result? Read and write operations so quick, they’d give The Flash a run for his money. This speed makes it a rockstar for things like caching data everyone’s trying to grab, keeping track of who’s logged in, crunching numbers as they happen, and even playing traffic cop for messages between different parts of your app.
Now, why bring Python into this speed-fest with redis-py
? Oh, let me count the ways (but not with ‘Firstly, Secondly,’ because that’s just not how we roll). For starters, getting Redis and Python to play nice together is smoother than a fresh jar of peanut butter; Python developers can weave Redis into their projects without breaking a sweat. Then there’s the sheer oomph it gives your application – we’re talking serious reductions in lag and a much snappier experience for your users. And to top it off, redis-py
speaks fluent Redis, supporting all its versatile data structures like strings, lists, sets, and hashes. So, it’s not just about raw speed; it’s about smart, flexible speed that gets along famously with Python.
III. Setting Up Redis and redis-py
Alright, eager beaver, let’s get your hands dirty! First things first, you’ll need a Redis server humming away. If you’re a fan of keeping things neat and tidy (and who isn’t?), Docker is your friend:
docker run -d -p 6379:6379 redis:latest
With Redis purring in the background, let’s grab the redis-py
package. Fire up your terminal and let pip do its magic:
pip install redis
And now, for the grand moment of connection! Here’s a little Python snippet to say ‘hello’ to your Redis server:
import redis
# Your very own Redis connection!
r = redis.Redis(host='localhost', port=6379, db=0)
# Let's teach Redis a new trick: storing 'Hello, Redis!' under the key 'mykey'
r.set('mykey', 'Hello, Redis!')
# And now, the grand reveal!
value = r.get('mykey')
print(value.decode('utf-8')) # Abracadabra! Output: Hello, Redis!
See? Easy peasy! You’ve just had your first conversation with Redis using redis-py
. Give yourself a pat on the back.
IV. Core Concepts and Data Structures in redis-py
Think of Redis as a Swiss Army knife for your data, equipped with a nifty set of tools – or data structures, if you want to get technical. Each one is a champ in its own right, ready for specific jobs:
- Strings: The bread and butter of Redis. Simple key-value pairs, like digital sticky notes. Super handy for caching little bits of info or keeping score (literally, with counters!).
r.set('page_views', 1) r.incr('page_views') # Someone else just visited! print(r.get('page_views').decode('utf-8')) # Output: 2 (Popular page, eh?)
- Lists: Your go-to for keeping things in order, like a digital to-do list or a queue at the post office (but way faster). Perfect for managing tasks that need doing one after another.
r.lpush('errands', 'buy_milk') r.lpush('errands', 'walk_the_redis_dog') # Important stuff first! next_errand = r.rpop('errands') # What's next on the agenda? print(next_errand.decode('utf-8')) # Output: buy_milk (The dog can wait... or can it?)
- Sets: Think of these as exclusive clubs where everyone is unique – no duplicates allowed! Brilliant for tracking unique visitors to your site or powering those ‘people who liked this also liked…’ features.
r.sadd('cool_users', 'user123') r.sadd('cool_users', 'user456') r.sadd('cool_users', 'user123') # Nope, still just one 'user123' in this cool club. print(r.smembers('cool_users')) # Output: {b'user456', b'user123'} (Order doesn't matter, just uniqueness!)
- Hashes: These are like little filing cabinets within Redis. You have a main key (the cabinet), and inside, you store multiple field-value pairs (folders and files). Just the ticket for organizing things like user profiles or detailed product info.
r.hset('user:goldfish_brain', 'name', 'Gary') r.hset('user:goldfish_brain', 'email', 'gary@example.com') print(r.hgetall('user:goldfish_brain')) # Output: {b'name': b'Gary', b'email': b'gary@example.com'} (All of Gary's deets!)
- Sorted Sets: Similar to Sets, but with a VIP twist – each member comes with a score! This lets you keep everything neatly ordered. Want to build a high-score leaderboard for your game or rank products by popularity? Sorted Sets are your MVP.
r.zadd('game_scores', {'PlayerOne': 5000}) r.zadd('game_scores', {'NoobMaster69': 9001}) # It's over 9000! print(r.zrange('game_scores', 0, -1, withscores=True)) # Output: [(b'PlayerOne', 5000.0), (b'NoobMaster69', 9001.0)] (Sorted by score, naturally)
V. Advanced Usage and Commercial Applications
Okay, you’ve mastered the basics. Ready to see redis-py
pull some serious rabbits out of its hat for the big leagues? Let’s dive into some pro-level commercial applications:
-
Caching Strategies (The Art of Not Annoying Your Database): Implement the ‘cache-aside’ pattern like a seasoned pro. This means Redis steps in front of your main database, taking the heat for frequently requested data. Slap an expiration time (TTL) on those cache entries, and you’ll keep things fresh without pestering your poor database for every little thing.
def get_shiny_product(product_id): # Is it already chilling in our Redis cache? product_from_cache = r.get(f'product:{product_id}') if product_from_cache: print("Found it in the cache! That was easy.") return product_from_cache.decode('utf-8') else: # Nope, gotta fetch it the old-fashioned (slower) way from the DB print("Cache miss! Off to the database we go...") # This is where you'd actually fetch from your database: # product_from_db = fetch_product_from_db(product_id) product_from_db = f"Super Shiny Product {product_id} Data" # Placeholder # Now, stash it in Redis for next time (for an hour, anyway) r.setex(f'product:{product_id}', 3600, product_from_db) return product_from_db
-
Session Management (Who Are You, Again?): Keep track of user sessions in Redis. It’s fast, scalable, and stops your app from having the memory of a goldfish when users click around.
-
Real-time Analytics (Knowing What’s Hot, Right Now): Use Redis to count clicks, track user shenanigans, and basically spy on everything happening in real-time. Hook this up to some fancy dashboards with WebSockets, and you’ll feel like you’re in a mission control room.
-
Message Queuing (Don’t Do Everything At Once!): Let Redis play postmaster with
redis-py
. It can act as a message broker for task queues (think Celery), helping to distribute workloads so your app doesn’t try to juggle too many chainsaws at once. -
Implementing Locks (Mine! No, Mine!): In the chaotic world of distributed systems, sometimes multiple processes try to grab the same cookie. Redis can act as the bouncer, using distributed locks to prevent digital fisticuffs (aka race conditions).
-
AI Applications (Making Your AI Even Smarter… and Faster): Redis is flexing its muscles in the AI arena too! Think vector storage for those fancy similarity searches, or LangCache for giving your AI a better memory with semantic caching. And speaking of making AI smarter, if you’re wrestling with turning messy, unstructured data into pristine, AI-ready gold, you might want to peek at what UndatasIO is cooking. They help streamline your RAG pipeline, making your AI applications quicker on the uptake and more on the money.
- Curious how to turn your data jumble into AI fuel? Learn More about UndatasIO.
-
Cluster Mode (More Power, Scotty!): When one Redis isn’t enough (because your app is that popular),
redis-py
can talk to a whole Redis Cluster. This means your data gets spread across multiple nodes, giving you awesome scalability and the ability to laugh in the face of (some) hardware failures.
VI. Performance Optimization
Want to make Redis go ‘vroom vroom’ even louder? Let’s fine-tune your redis-py
setup and squeeze every last millisecond of performance out of it:
-
Connection Pooling (Stop Making New Friends All The Time): Constantly opening and closing connections to Redis is like repeatedly introducing yourself at a party – tiring and inefficient. Connection pooling reuses existing connections, which is much kinder on resources.
# Create a pool of up to 10 connections pool = redis.ConnectionPool(host='localhost', port=6379, db=0, max_connections=10) r_pooled = redis.Redis(connection_pool=pool) # Renamed to avoid conflict if 'r' is global # Now 'r_pooled' will just grab a ready-to-go connection from the pool!
-
Pipelining (Sending Your Shopping List All At Once): Instead of sending commands to Redis one by one (like asking for milk, then eggs, then bread separately), pipelining lets you bundle them up. Fewer trips mean faster results!
# Assuming 'r_pooled' or your preferred Redis connection object pipe = r_pooled.pipeline() pipe.set('item1', 'spam') pipe.set('item2', 'more_spam') pipe.execute() # Bam! All done in one go.
-
Monitoring and Tuning (Playing Detective): Keep an eye on things! Use the Redis
MONITOR
command (it’s like a live feed of Redis gossip) and dive into performance metrics. This helps you spot any sluggish spots and tweak your configuration for peak awesomeness.And hey, since we’re on the topic of peak awesomeness, especially for AI, remember that your AI is only as good as the data you feed it. You wouldn’t put regular unleaded in a Ferrari, right? While some tools give you the basic nuts and bolts for data extraction, if you’re serious about getting AI-ready, structured data from the wild west of unstructured files, UndatasIO is like the high-octane fuel and pit crew for your AI models, ensuring they run smoother and smarter.
VII. Redis Alternatives and Considerations
Now, as much as we’ve been singing Redis’s praises (and it deserves a good choir!), it’s fair to say it’s not the only speedy Gonzales in the data store town. You’ve got other options like Memcached, which is another popular choice for caching, or various cloud-based in-memory gizmos. And with those recent licensing shake-ups we mentioned, a new challenger named Valkey has entered the arena – a community-driven fork aiming to carry the torch.
So, what’s a savvy developer to do? Well, it’s all about playing matchmaker. Take a good, hard look at what your project really needs, peek at your budget (always a party pooper, that one), and then pick the tool that makes your heart (and your app) sing. Redis might be your soulmate, or perhaps one of these other bright sparks will catch your eye.
VIII. Best Practices and Anti-Patterns
To keep your Redis journey smooth and steer clear of digital potholes, here are a few golden rules and things that make experienced devs go ‘tsk tsk’:
- Don’t Treat Redis Like a Data Landfill: Resist the urge to stuff gigantic blobs of data into Redis. It’s built for speed with smaller, frequently accessed items. Think sprinters, not sumo wrestlers.
- Production-Grade Setup is Key: When you’re ready to go live, don’t just wing it. Configure Redis with care for your production environment. This isn’t your dev playground anymore, Toto!
- Data Durability – Don’t Lose Your Precious Bytes! Remember, Redis is primarily in-memory. If you don’t want your data to vanish in a puff of smoke if the server hiccups, get familiar with persistence options like RDB (snapshots) or AOF (logging every write). Your future self will thank you.
- Lock It Down! Leaving your Redis instance wide open to the internet is like putting a “Free Kittens & Data” sign on your door. Implement robust security measures. Seriously. Don’t be that person.
IX. Conclusion
So there you have it! Redis and its Python pal, redis-py
, are quite the power couple, ready to inject some serious pep into your applications, help them scale like a champ, and generally make them more reliable in the rough-and-tumble world of commercial software. By getting chummy with the right caching tactics, data structures, and those nifty optimization tricks, you’re well on your way to making Redis sing. When set up with a bit of smarts and a team that knows its way around, Redis isn’t just a tool; it’s a game-changer for your business.
X. Call to Action
Feeling revved up and ready to roll? Go on, dive in and start sprinkling some redis-py
magic into your projects today! We’ve even got a free checklist to help you nail those Redis caching best practices – consider it your treasure map supplement. Got tales from your own Redis adventures, or burning questions? Drop them in the comments below; we’d love to hear them! And if you’re looking to really hit the accelerator, our Redis hosting and consulting services are here to help you zoom.
P.S. Speaking of leveling up, especially if you’re dabbling in the ever-exciting world of AI, transforming that messy pile of unstructured data into pure, unadulterated AI gold is crucial. If that sounds like a quest you’re on, take a gander at UndatasIO. They’re wizards at turning data chaos into AI-ready assets. Give it a whirl!.
📖See Also
- In-depth Review of Mistral OCR A PDF Parsing Powerhouse Tailored for the AI Era
- Assessment-Unveiled-The-True-Capabilities-of-Fireworks-AI
- Evaluation-of-Chunkrai-Platform-Unraveling-Its-Capabilities-and-Limitations
- IBM-Docling-s-Upgrade-A-Fresh-Assessment-of-Intelligent-Document-Processing-Capabilities
- Is-SmolDocling-256M-an-OCR-Miracle-or-Just-a-Pretty-Face-An-In-depth-Review-Reveals-All
- Can-Undatasio-Really-Deliver-Superior-PDF-Parsing-Quality-Sample-Based-Evidence-Speaks
Subscribe to Our Newsletter
Get the latest updates and exclusive content delivered straight to your inbox