Mastering Unstructured Data with Python: A Practical Guide
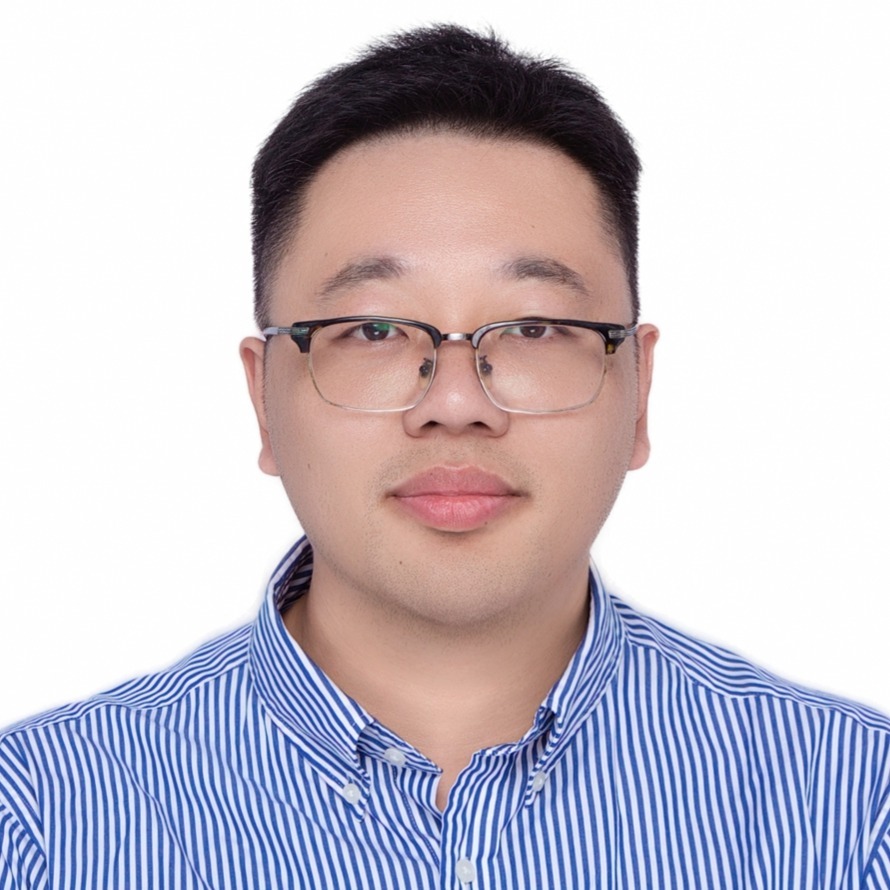
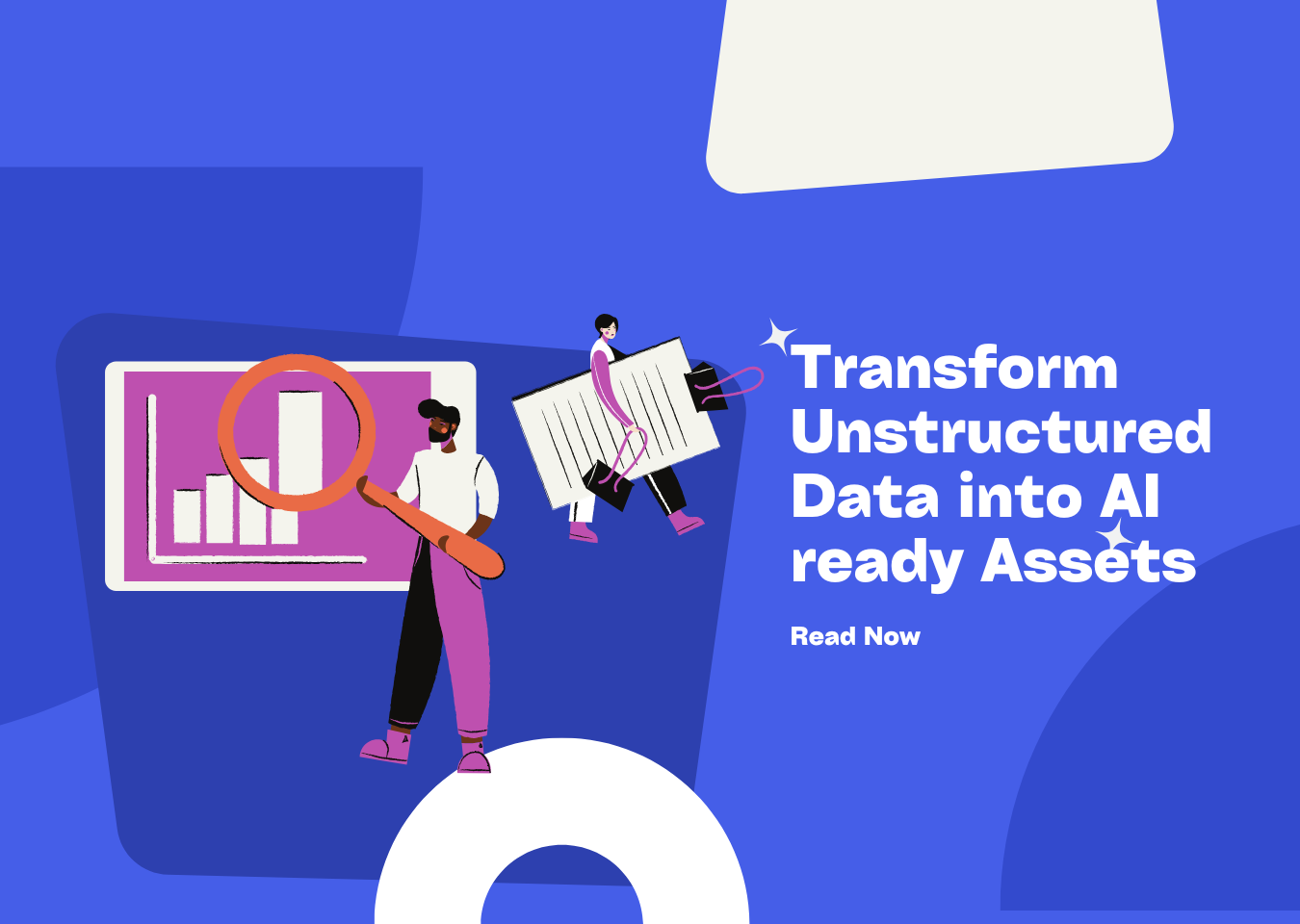
Ever feel like you’re drowning in digital paperwork? PDFs, Word docs, emails, web pages… it’s a tidal wave of information! The kicker? Most of this stuff – estimates say a whopping 80-90% – is what tech folks call “unstructured.” Think of it like your digital junk drawer: potentially useful things are in there, but good luck finding anything specific without rummaging for ages.
Trying to pull useful info out of this digital chaos is usually a headache. Doing it by hand? Slow, boring, and about as scalable as counting grains of sand. This digital mess makes it tough for computers (and let’s be honest, us humans) to make sense of it all.
But fear not, data wranglers! There’s a nifty Python tool called unstructured
ready to swoop in and save the day. It’s like a super-smart assistant that can read almost anything you throw at it, tidy it up, and hand you back the important bits in a neat, organized pile. Forget manual drudgery; this open-source hero helps you finally understand what all those files are trying to tell you.
So, why should you care? Because hidden in that digital mess is gold! We’re talking customer feedback in emails, key facts buried in reports, vital stats on web pages… you name it. If you’re building AI apps or working with fancy systems like RAG (Retrieval-Augmented Generation), getting this data out cleanly is crucial. (And psst… if you need to do this at industrial scale, companies like UndatasIO are building tools specifically for this kind of heavy lifting, turning messy data into AI fuel.)
Okay, But What Is This ‘unstructured’ Thing, Really?
Glad you asked! unstructured
is an open-source Python library – basically, a free toolkit for your code – built specifically to tackle messy text and image documents. It dives into PDFs, Word files, HTML pages, and more, parses them (reads them intelligently), cleans up the gunk, and structures the valuable information. Think of it as translating document gibberish into something your computer can actually understand and use.
One of its superpowers? It plays nice with other popular tools, especially friends like LangChain (a framework for building AI language applications). This means you can easily slot unstructured
into bigger projects, feeding clean data straight into powerful AI models. Plus, getting it is easy – it’s readily available for anyone with Python.
(While unstructured
is awesome for getting started and many common tasks, keep in mind that for super complex, enterprise-level data transformations, solutions like UndatasIO offer a more complete, managed pipeline.)
Let’s Get It Installed! (Don’t Worry, It’s Easy)
Ready to give it a whirl? First, make sure you have Python installed (if you’re reading this, you probably do!). Then, open your terminal or command prompt and type:
pip install unstructured
Boom! That gets you the basic package. Now, unstructured
is clever, but it needs a little help to understand specific file types perfectly. If you plan on tackling PDFs (a common culprit!), you’ll need an extra bit:
pip install unstructured[pdf]
Want to be prepared for anything? Go full Rambo with:
pip install unstructured[all-docs]
This installs everything needed for all the document types it supports. A few seconds of installation for hours of saved headaches? Yes, please!
Basic Training: Making a PDF Spill Its Guts
Alright, let’s get our hands dirty with a simple example. Suppose you have a PDF named example.pdf
. Here’s how you can magically pull the text out:
from unstructured.partition.auto import partition
# Tell it which file you want to dissect
filename = "example.pdf"
# Let the magic happen!
elements = partition(filename=filename)
# Now, let's see what it found
for element in elements:
print(element.text)
print("---") # Just adding a separator for clarity
See that partition
function? It’s the star of the show. It automatically figures out it’s a PDF and uses the right tools to break it down into logical chunks – things like titles, paragraphs, maybe even lists or table bits. Then, you just loop through these elements
and grab the .text
. Simple, right? From messy PDF to clean text, just like that!
It’s Not Just PDFs! Word Docs & HTML Fear It Too
unstructured
isn’t a one-trick pony. Got Word documents (.docx
) or HTML files cluttering up your hard drive? No problem!
Wrangling a Word Document:
from unstructured.partition.docx import partition_docx
filename = "example.docx" # Your Word doc here
elements = partition_docx(filename=filename)
for element in elements:
print(element.text)
print("---")
Taming an HTML File:
from unstructured.partition.html import partition_html
filename = "example.html" # Your HTML file here
elements = partition_html(filename=filename)
for element in elements:
print(element.text)
print("---")
Notice a pattern? While the specific function might change slightly (partition_docx
, partition_html
), the basic idea is the same: point it at the file, let it partition, and loop through the results. It even tries its best to handle things inside those documents, like images or tables, though your mileage may vary depending on complexity.
Level Up: Fancy Moves and API Magic
Okay, so basic text grabbing is cool, but unstructured
has more tricks up its sleeve. You can tweak how it partitions documents, adjust settings for finer control, and sometimes get structured data from tables.
Need even more power or don’t want to install everything locally? They offer an API! You can send your file to their servers, and they’ll process it and send back the structured results. Handy for web apps or bigger systems.
# This is a conceptual example - you'd need an API key!
from unstructured.partition.auto import partition
import requests # Needs 'pip install requests'
# Replace with your actual API key and file
api_key = "YOUR_API_KEY"
file_path = "example.pdf"
response = requests.post(
"https://api.unstructured.io/v1/general/",
files={"files": (file_path, open(file_path, "rb"))},
headers={"unstructured-api-key": api_key}, # Use the correct header
)
if response.status_code == 200:
elements = response.json()
for element in elements:
# API response structure might differ slightly
print(element.get("text", "No text found"))
print("---")
else:
print(f"API call failed: {response.status_code} - {response.text}")
Using the API opens up possibilities for building cloud-powered apps that can chew through documents from anywhere.
Playing Nice with LangChain
If you’re building applications with Large Language Models (LLMs), you’ve probably heard of LangChain. Good news! unstructured
fits into LangChain like a glove. LangChain uses unstructured
behind the scenes to load and prepare your documents, making it super easy to feed your messy files to your hungry AI.
Here’s a taste:
from langchain_community.document_loaders import UnstructuredFileLoader # Note the import path might vary slightly depending on your langchain version
loader = UnstructuredFileLoader("example.pdf")
documents = loader.load()
# LangChain often puts the content in 'page_content'
print(documents[0].page_content)
With this integration, building sophisticated Q&A systems over your documents, summarizing reports, or other cool NLP tasks becomes much, much smoother.
Now, if you find yourself building really complex AI pipelines with LangChain and need more than just basic parsing – maybe advanced cleaning, metadata enrichment, or ensuring data is perfectly primed for your specific AI model – that’s where tools like UndatasIO really shine. They often offer features beyond what standalone unstructured
or simpler parsers provide, aiming for that truly “AI-ready” data state.
Stop Drowning, Start Structuring!
So there you have it. The unstructured
module is your secret weapon against the chaos of unstructured data. It’s relatively easy to use, handles a bunch of file types, and plugs right into the AI tools you might already be using (like LangChain). Whether you’re trying to feed data to a machine learning model, archive documents intelligently, or just finally figure out what’s in that mountain of PDFs, unstructured
is worth a look.
Think of all the time you’ll save not having to manually copy-paste or decipher messy documents! It turns a painful chore into a manageable (and sometimes even fun!) task.
Go ahead, dive into the official unstructured
documentation, try out the examples, and see how you can use it to make sense of your own digital world. There’s a whole community around it, so help is usually easy to find.
And remember, if your data challenges grow and you need an enterprise-grade solution to turn that unstructured data into perfectly tuned AI-ready assets, check out platforms like UndatasIO. They build on these concepts to offer even more power.
Happy structuring!
📖See Also
- In-depth Review of Mistral OCR A PDF Parsing Powerhouse Tailored for the AI Era
- Assessment-Unveiled-The-True-Capabilities-of-Fireworks-AI
- Evaluation-of-Chunkrai-Platform-Unraveling-Its-Capabilities-and-Limitations
- IBM-Docling-s-Upgrade-A-Fresh-Assessment-of-Intelligent-Document-Processing-Capabilities
- Is-SmolDocling-256M-an-OCR-Miracle-or-Just-a-Pretty-Face-An-In-depth-Review-Reveals-All
- Can-Undatasio-Really-Deliver-Superior-PDF-Parsing-Quality-Sample-Based-Evidence-Speaks
Subscribe to Our Newsletter
Get the latest updates and exclusive content delivered straight to your inbox